How to use?
Pair a number
Connect your or any other number via QR code for testing
Test API methods
It’s take only 1 Minute to Get Started using the our easy-to-use Developer Tool
Setup your Webhook
Select and configure Webhook on any event in WhatsApp to send and receive messages
What is Whapi.Cloud?
Straightforward and powerful! Whapi.Cloud is an intuitive API that enables you to seamlessly connect your business with WhatsApp.
With Whapi.Cloud, you can focus on what really matters – growing your business.
Enjoy endless integration possibilities, such as building support bots, scheduling appointments, sending WhatsApp notifications, and so much more.
Plus, monitor everything effortlessly with webhooks. Getting started has never been easier!
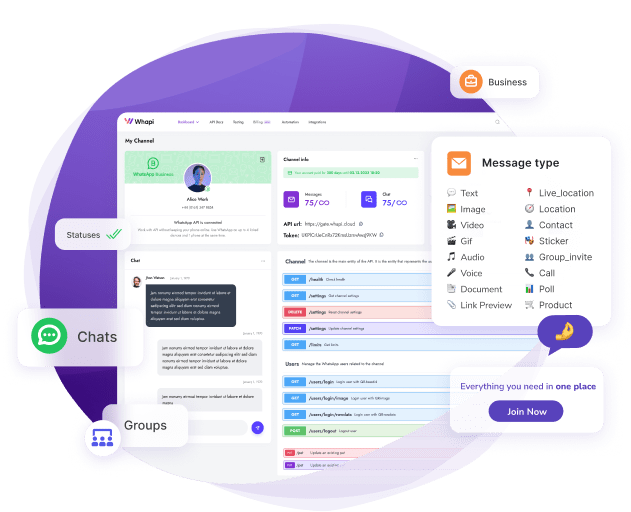
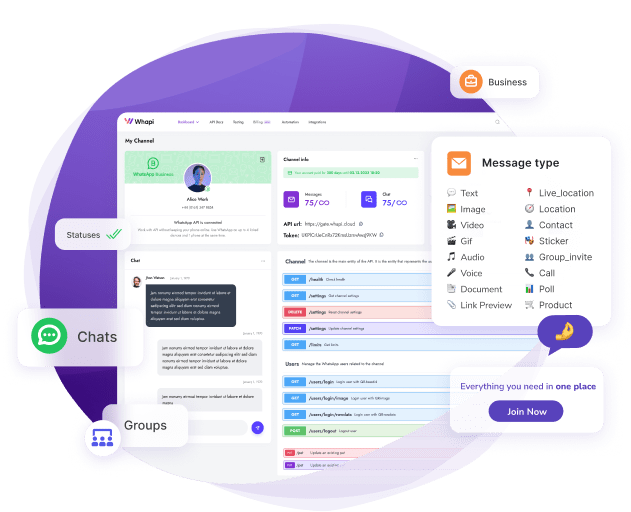
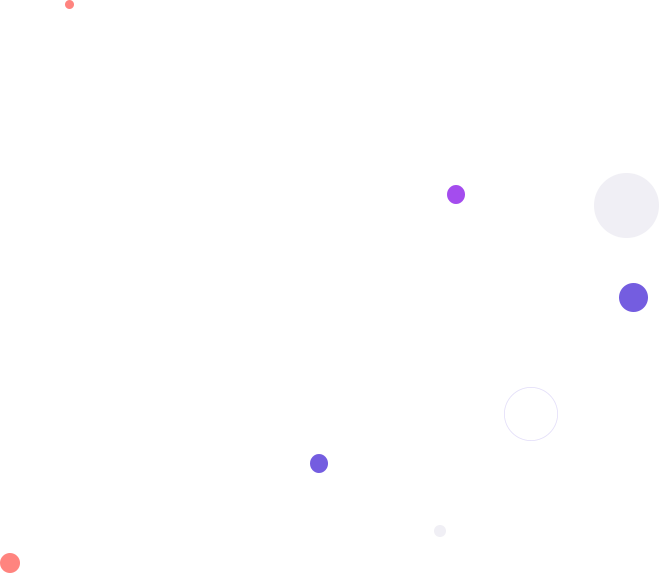
Check out the WhatsApp API
We regularly update new features to improve your experience. If you are looking for a missing feature, please contact our support team.
WhatsApp API PHP SDK Documentation
Installation
composer require whapi-cloud/whatsapp-api-sdk-php
Initial Setup
use OpenAPI\Client\Api\MessagesApi;
use OpenAPI\Client\Configuration;
// Initialize the client
$config = OpenAPI\Client\Configuration::getDefaultConfiguration()
->setApiKey('token', "your_token")
->setAccessToken("your_token");
$apiInstance = new OpenAPI\Client\Api\MessagesApi(
new GuzzleHttp\Client(),
$config
);
Messages API
Sending Text Messages
// Create text message object
$sender_text = new \OpenAPI\Client\Model\SenderText();
$sender_text->setTo('13016789891'); // Include country code
$sender_text->setBody('Your message here'); // Message content
// Optional parameters
$sender_text->setEphemeral(3600); // Message visibility time in seconds
$sender_text->setViewOnce(true); // View once setting
$sender_text->setTypingTime(5.0); // Typing simulation duration
$sender_text->setNoLinkPreview(false); // Enable/disable link previews
// Send the message
$result = $apiInstance->sendMessageText($sender_text);
print_r($result);
Sending Images with Captions
// Create image message object
$sender_image = new \OpenAPI\Client\Model\SenderImage();
$sender_image->setTo('13016789891'); // Recipient's number
$sender_image->setCaption('Image caption');
$sender_image->setMedia('https://example.com/image.jpg');
// Send the image
$result = $apiInstance->sendMessageImage($sender_image);
print_r($result);
Retrieving Messages
Get Multiple Messages
// Parameters
$count = 100; // Number of messages to retrieve
$offset = 0; // Pagination offset
// Get messages
$result = $apiInstance->getMessages($count, $offset);
print_r($result);
Get Single Message
// Get message by ID
$message_id = "your_message_id";
$result = $apiInstance->getMessage($message_id);
print_r($result);
WhatsAppp Groups API
Creating Groups
$apiInstance = new OpenAPI\Client\Api\GroupsApi(
new GuzzleHttp\Client(),
$config
);
// Create group request
$create_group_request = [
"subject" => "Group Name",
"participants" => [
"13016789891",
"13016789892"
]
];
// Create the group
$result = $apiInstance->createGroup($create_group_request);
print_r($result);
Important Notes
- Phone Numbers:
- Always include country code (e.g., '13016789891' for US number)
- Don't use special characters or spaces
- Media URLs:
- Must be publicly accessible
- Support common image formats (JPEG, PNG, etc.)
- Message Options:
- Ephemeral: Time in seconds before message disappears
- ViewOnce: Message can only be viewed once
- TypingTime: Simulates natural typing delay
Error Handling
try {
$result = $apiInstance->sendMessageText($sender_text);
print_r($result);
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
- Avoid sending too many messages in quick succession
- Implement proper error handling
- Store and handle message IDs for tracking
- Regular monitoring of API responses
- Maintain proper logging for debugging